I had the privilege of being one of the 200 or so attending a Google Dev Group meeting in which a few of Google’s Android Developer Advocates gave a couple of talks as well as had the AOL development team present a demo of their work which will soon be published in the market. The meeting was held in an auditorium of AOL’s campus which was pretty nice and could hold easily hold the 200 attendees. The Google guys bought tons of pizza for everyone. A big thanks to both AOL for the room and Google for the food. =D If you follow the meeting link, you can see the pictures taken at that event.
The first speaker was David Chandler who is the primary developer behind the WebView widget and is the primary force behind GWT. He was in town for the FCC’s meeting on Accessibility in Mobile Apps and the Mobile Web and agreed to be one of the speakers for this little shindig. He talked about WebView apps and how many of them are designed on-the-cheap as a way to target several different mobile ecosystems with one app. He pointed out how many of them are also designed to look like an iOS app… which is incongruous and confusing for an Android user and basically to “don’t do that”. I learned that AsyncTasks are great for background tasks, but not for a task that may get killed mid-run if the device orientation changes as they will get cancelled (use a Service instead). Spring Android is an open source project with a REST encapsulation set of classes to make those kinds of calls easy inside Android. Building a service around Spring Android would be the best practice, but you can put it anywhere you like, even inside an AsyncTask.
Android 4.1 (JellyBean) introduced a new set of notifications, with a much larger size and buttons and even a short list. One of the problems with this new set was a fairly hidden feature of the expandable notification (hidden because it isn’t really discoverable): some notifications can be expanded if you use two fingers to “pull down” which would expand it. Not really something hinted at visually and, for people like me, impossible to discover because I am usually using the phone one handed, preventing the use of two finger gestures most times.
The Google I/O 2012 App demonstrating many of the new features is still available on Google Play, but not for much longer. Source code for the app will be available for a long time to come and can be seen/downloaded from here. Demonstrated features include: the new notifications, ICS calendar integration, GCM (successor to C2DM – push notification from web server), Android Beam (using the NFC), and more.
David then went on to discuss the FCC meeting on Accessibility and proceeded to talk about various quick and easy ways to vastly improve accessibility of an app for the visually impaired. He gave a short example of a slider bar and how, even with text to speech enabled with various text being read as you operated it, the UX is quite cumbersome. Supplementing the slider bar with simple + and – buttons to move the slider up and down did not add much complexity, but made the task of properly setting the value of the slider so much easier by just using those simple buttons instead.
The AOL Android development team gave their demonstration next and they showed off their fairly sophisticated magazine reader app that had multiple call outs, gesture actions, vertical page scrolling, and even content drill down based on hot spots defined in the xml content. The thing I found most fascinating was their ability to hook up a device directly to the screen projector and show off the app that way (at least for the tablet, their phone could not hook up). When I inquired about that ability after the event, they said it was a Motorola Xoom with an HDMI port. After I got home, I immediately checked my ASUS tablet for such a port and found it has a microHDMI output. The Samsung Galaxy S III handset also has one, so I may need to get one of those kinds of phones the next time I need to do a presentation with a handset. AOL On is a new Google TV app that will add feature rich content from AOL as a set of new channels for you to watch on your Google TV, including HuffLive. While I technically found the apps presented impressive, I don’t typically read the magazines they would be delivering nor do I have a Google TV. Others will have to comment about them once they get published (which should be soon, this was the first time anyone outside AOL got to see anything about them).
Adam Koch and Roman Nurik came down from Google’s NYC office to give a micro version of their Google IO presentation about the features of JellyBean (4.1), best practices for UI design, and how best to design and develop with the Nexus 7… which is quirky because of it’s default portrait mode instead of landscape, being 7″ with a “tvdpi” of 213dpi, and only a front facing camera. They talked about UI design, UX should be “butter” (smooth — opposite of “janky”), making sure we have “hardware acceleration” option turned on in our apps and that we should all review the design guidelines with regards to how the new Up button behaves (which is the app icon) vs. the Back button which has been around forever. A special note was said to pay attention to the new activity attribute parentActivityName and the TaskStackBuilder class.
Adam asked at one point during his talk if anyone created a “universal app” which targeted any and all screen sizes. As far as I know, I was the only one to raise my hand. He asked me about the challenges I faced and I mentioned all the different layout folders and value folders necessary to create such apps. During Roman’s talk about best practices for UI design in helping to support “universal screen apps”, he asked the audience to see how many were using the “smallest width” layout filter (i.e. layout-sw600 folder for 7″ tablet layouts as apposed to “layout” for handsets and “layout-sw720″ for 10” tablets). When Roman saw that I was the only one with my hand up, he commented “Only one? That’s very disappointing. You all should be using it”. It made me realize that my development process and experience is not only valuable, but rare, too (around here, at least).
All in all, it was a very informative 2 hour set of talks and demonstrations and I appreciated every minute of it. Thanks again to Google and AOL for sponsoring the event and presenting something like that. Very cool. =)
As I left the parking garage at AOL’s campus, I was greeting with this warning sign.
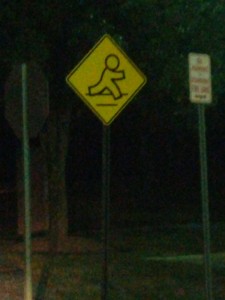
Crosswalk warning sign at AOL