The Android notification bar has been white for a very long time. A great many apps that put up icons inside this bar create black icons with transparencies so they appear black on white when viewed against this white notification bar. Unfortunately, with Android 2.3.3+ and 3.0+, the notification bar is now black. Black icons on black bars do not show up well at all.
I highly advise against creating separate icons for the different versions and instead offer the tip of creating a white background for your black icons. In my apps that require a notification icon, I gave the icons a round, white background that used the alpha channel to fade the white from the center to the edge. The effect is that on the older, white notification bars, no difference is detected since white fading into white is the same thing as nothing at all. On a black background, however, the effect is that my black icon is visible within a white circle that fades to black, making it look like a modern, 3D rounded icon.
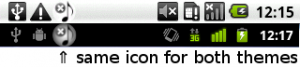
Personally, I detest the current state of my 2.3.4 phone’s notification colors since most of the apps currently use black or dark grey notification icons (including stock apps) and as such makes it nearly impossible to see them at a glance when checking my phone for messages out in bright daylight. Whomever’s bright idea it was to push this change out to phones that have been using and expecting white notification bars for years now should be given a painful wedgie!